Bootstrap Components
This is a quick showcase of some of the main Bootstrap components that come with this theme. Theme uses React Bootstrap plugin which extends Bootstrap framework and makes using Bootstrap in React easy.
This theme uses React Bootstrap plugin which extends Bootstrap framework and makes using Bootstrap in React easy. Read more about React Bootstrap here.
Alert
Provide contextual feedback messages for typical user actions with the handful of available and flexible alert messages. See the React Bootstrap documentation for more details.
import { Alert } from 'react-bootstrap'
const Component = () => {
return (
<Alert variant="primary">This is a primary alert—check it out!</Alert>
)
}
export default Component
Badge
Small count and labeling component. See the React Bootstrap documentation for more details.
Add -light
suffix to the class name to get lighter tones on badges.
Example heading New
Example heading New
Example heading New
Example heading New
Example heading New
import { Badge } from 'react-bootstrap'
export default () => {
return (
<Badge bg="primary">
primary
</Badge>
<Badge
bg="secondary-light"
text="secondary"
pill
>
secondary
</Badge>
)
}
Card
Bootstrap’s cards provide a flexible and extensible content container with multiple variants and options.. See the React Bootstrap documentation for more details.
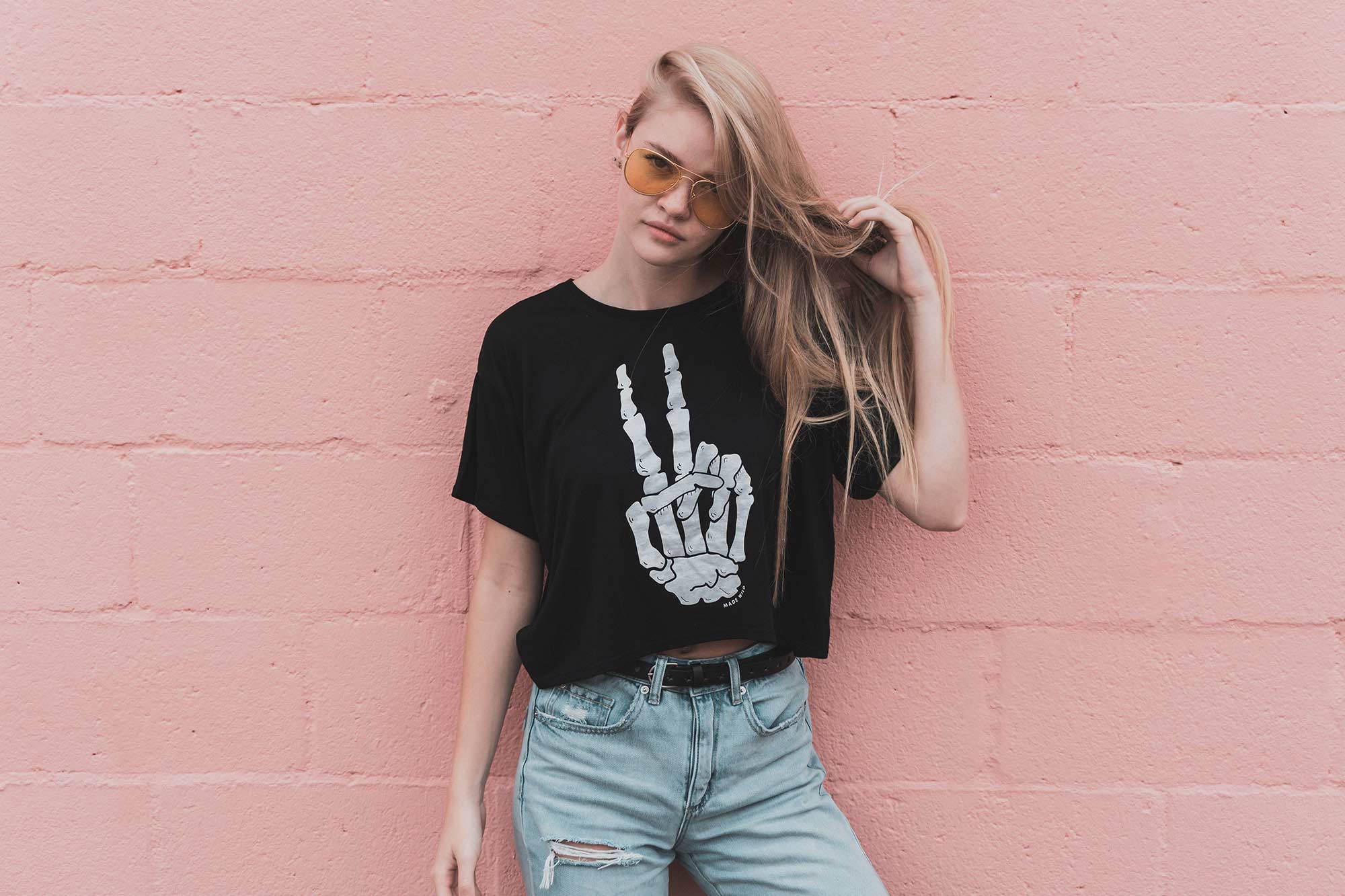
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereimport { Card, Button } from 'react-bootstrap'
const Component = () => {
return (
<Card>
<Card.Img top src="/img/photo/ian-dooley-347962-unsplash.jpg" alt="Card image cap" />
<Card.Body>
<Card.Title tag="h4">Card title</Card.Title>
<Card.Text>Some quick example text to build on the card title and make up the bulk of the card's content.</Card.Text>
<Button href="#" variant="dark">Go somewhere</Button>
</Card.Body>
</Card>
)
}
export default Component
Dropdowns
Toggle contextual overlays for displaying lists of links and more with the Bootstrap dropdown plugin. See the React Bootstrap documentationfor more details.
import { Dropdown } from 'react-bootstrap'
export default () => {
return (
<Dropdown drop="down" className="d-inline-block">
<Dropdown.Toggle variant="outline-primary">Default</Dropdown.Toggle>
<Dropdown.Menu>
<Dropdown.Header className="fw-normal">
Dropdown header
</Dropdown.Header>
<Dropdown.Item href="#">Action</Dropdown.Item>
<Dropdown.Item href="#">Another action</Dropdown.Item>
<Dropdown.Item href="#">Something else here</Dropdown.Item>
<Dropdown.Divider />
<Dropdown.Item href="#">Something else here</Dropdown.Item>
</Dropdown.Menu>
</Dropdown>
)
}
Forms
Examples and usage guidelines for form control styles, layout options, and custom components for creating a wide variety of forms. See the React Bootstrap documentation for more details.
Form Group
Input Sizes
Input Group
Custom Inputs
import { Form } from 'react-bootstrap'
export default () => {
return (
<Form>
<div className="mb-4>
<Form.Label htmlFor="exampleInputEmail1">Email address</Form.Label>
<Form.Control id="exampleInputEmail1" type="email" aria-describedby="emailHelp" placeholder="Enter email" />
<Form.Text id="emailHelp">We'll never share your email with anyone else.</Form.Text>
</div>
</Form>
)
}
List Group
List groups are a flexible and powerful component for displaying a series of content. Modify and extend them to support just about any content within. See the React Bootstrap documentation for more details.
import { ListGroup } from 'react-bootstrap'
export default () => {
return (
<ListGroup>
<ListGroup.Item>Cras justo odio</ListGroup.Item>
<ListGroup.Item>Dapibus ac facilisis in</ListGroup.Item>
<ListGroup.Item>Morbi leo risus</ListGroup.Item>
<ListGroup.Item>Porta ac consectetur ac</ListGroup.Item>
<ListGroup.Item>Vestibulum at eros</ListGroup.Item>
</ListGroup>
)
}
Modal
Use Bootstrap’s JavaScript modal plugin to add dialogs to your site for lightboxes, user notifications, or completely custom content. See the React Bootstrap documentation for more details.
import { Modal, Button } from 'react-bootstrap'
export default () => {
const [modal, setModal] = React.useState(false)
const onClick = () => {
setModal(!modal)
}
return (
<>
<Button onClick={onClick}>Launch demo modal</Button>
<Modal show={modal} onHide={onClick}>
<Modal.Header className="text-center">
<Modal.Title className="w-100" as="h2">
Modal heading
</Modal.Title>
<Button
variant="dark"
className="close modal-close p-0"
onClick={onClick}
>
<Icon
icon="close-1"
className="w-100 h-100 svg-icon-light align-middle"
/>
</Button>
</Modal.Header>
<Modal.Body>
<p className="text-muted">
<strong>Pellentesque habitant morbi tristique</strong> senectus et
netus et malesuada fames ac turpis egestas. Vestibulum tortor
quam, feugiat vitae, ultricies eget, tempor sit amet, ante. Donec
eu libero sit amet quam egestas semper.{" "}
<em>Aenean ultricies mi vitae est.</em> Mauris placerat eleifend
leo. Quisque sit amet est et sapien ullamcorper pharetra.
Vestibulum erat wisi, condimentum sed, <code>commodo vitae</code>,
ornare sit amet, wisi. Aenean fermentum, elit eget tincidunt
condimentum, eros ipsum rutrum orci, sagittis tempus lacus enim ac
dui. <a href="#">Donec non enim</a> in turpis pulvinar facilisis.
Ut felis.
</p>
</Modal.Body>
<Modal.Footer className="justify-content-center">
<Button variant="outline-dark" onClick={onClick}>
Close
</Button>
<Button onClick={onClick} variant="dark">
Save changes
</Button>
</Modal.Footer>
</Modal>
<>
)
}
Pagination
Pagination to indicate a series of related content exists across multiple pages. See the React Bootstrap documentation for more details.
import { Pagination } from 'react-bootstrap'
export default () => {
return (
<Pagination aria-label="Page navigation example">
<Pagination.First href="#">Previous</Pagination.First>
<Pagination.Item active href="#">
1
</Pagination.Item>
<Pagination.Item href="#">2</Pagination.Item>
<Pagination.Item href="#">3</Pagination.Item>
<Pagination.Item href="#">4</Pagination.Item>
<Pagination.Item href="#">5</Pagination.Item>
<Pagination.Next href="#">Next</Pagination.Next>
</Pagination>
)
}
Progress
Bootstrap custom progress bars featuring support for stacked bars, animated backgrounds, and text labels. See the React Bootstrap documentation for more details.
import { ProgressBar } from 'react-bootstrap'
export default () => {
return (
<>
<ProgressBar now={25} variant="primary" />
<ProgressBar now={50} variant="info" />
<ProgressBar now={75} variant="gray-600" />
<ProgressBar now={100} variant="dark" />
</>
)
}
Tooltips
Custom Bootstrap tooltips with CSS and JavaScript using CSS3 for animations and data-attributes for local title storage. See the React Bootstrap documentation for more details.
import { Button, Tooltip, OverlayTrigger } from 'react-bootstrap'
export default () => {
return (
<>
{["top", "right", "bottom", "left"].map((placement) => (
<OverlayTrigger
key={placement}
placement={placement}
overlay={
<Tooltip id={`tooltip-${placement}`}>
Tooltip on <strong>{placement}</strong>.
</Tooltip>
}
>
<Button variant="outline-primary" className="mb-1 me-1">
Tooltip on {placement}
</Button>
</OverlayTrigger>
))}
</>
)
}